原文:Python Parse JSON – How to Read a JSON File,作者:Jessica Wilkins
JSON(JavaScript Object Notation 对象表示法)是一种流行的结构化数据的方式。它被用来在网络应用程序和服务器之间交换信息。但是如何在 Python 中读取一个 JSON 文件呢?
在这篇文章中,我将向你展示如何使用 json.loads()
和 json.load()
方法来解析和读取 JSON 文件和字符串。
JSON 语法
在我们解析和读取 JSON 文件之前,我们首先需要了解基本的语法。JSON 的语法看起来像一个带有键值对的 JavaScript 对象字面。
这是一个 freeCodeCamp 的 JSON 数据的例子。
{
"organization": "freeCodeCamp",
"website": "https://www.freecodecamp.org/",
"formed": 2014,
"founder": "Quincy Larson",
"certifications": [
{
"name": "Responsive Web Design",
"courses": [
"HTML",
"CSS"
]
},
{
"name": "JavaScript Algorithms and Data Structures",
"courses": [
"JavaScript"
]
},
{
"name": "Front End Development Libraries",
"courses": [
"Bootstrap",
"jQuery",
"Sass",
"React",
"Redux"
]
},
{
"name": "Data Visualization",
"courses": [
"D3"
]
},
{
"name": "Relational Database Course",
"courses": [
"Linux",
"SQL",
"PostgreSQL",
"Bash Scripting",
"Git and GitHub",
"Nano"
]
},
{
"name": "Back End Development and APIs",
"courses": [
"MongoDB",
"Express",
"Node",
"NPM"
]
},
{
"name": "Quality Assurance",
"courses": [
"Testing with Chai",
"Express",
"Node"
]
},
{
"name": "Scientific Computing with Python",
"courses": [
"Python"
]
},
{
"name": "Data Analysis with Python",
"courses": [
"Numpy",
"Pandas",
"Matplotlib",
"Seaborn"
]
},
{
"name": "Information Security",
"courses": [
"HelmetJS"
]
},
{
"name": "Machine Learning with Python",
"courses": [
"Machine Learning",
"TensorFlow"
]
}
]
}
如何在 Python 中解析一个 JSON 字符串
Python 有一个内置的模块,允许你处理 JSON 数据。在你的文件的顶部,你需要导入 json
模块。
import json
如果你需要解析一个返回字典的 JSON 字符串,那么你可以使用 json.loads()
方法。
import json
# assigns a JSON string to a variable called jess
jess = '{"name": "Jessica Wilkins", "hobbies": ["music", "watching TV", "hanging out with friends"]}'
# parses the data and assigns it to a variable called jess_dict
jess_dict = json.loads(jess)
# Printed output: {"name": "Jessica Wilkins", "hobbies": ["music", "watching TV", "hanging out with friends"]}
print(jess_dict)
如何在 Python 中解析和读取一个 JSON 文件
在这个例子中,我们有一个叫做 fcc.json
的 JSON 文件,它保存了前面关于 freeCodeCamp 所提供的课程的相同数据。
如果我们想读取这个文件,我们首先需要使用 Python 内置的 open()
函数,其模式为读取。我们使用 with
关键字来确保文件被正确关闭。
with open('fcc.json', 'r') as fcc_file:
如果文件不能被打开,那么我们将收到一个 OSError。例如,如果我拼错了 fcc.json
的文件名,将出现 "FileNotFoundError "。

然后我们可以使用 json.load()
方法解析该文件,并将其分配给一个名为 fcc_data
的变量。
fcc_data = json.load(fcc_file)
最后一步将是打印结果。
print(fcc_data)
完整的代码是这样的:
import json
with open('fcc.json', 'r') as fcc_file:
fcc_data = json.load(fcc_file)
print(fcc_data)
如何在 Python 中更好地打印 JSON 数据
如果我们检查打印的数据,那么我们应该看到,JSON 数据全部打印在一行上。

但这可能很难阅读。为了解决这个问题,我们可以使用 json.dumps()
方法,其参数为 indent
。
在这个例子中,我们将缩进 4 个空格,以更容易阅读的格式打印数据。
print(json.dumps(fcc_data, indent=4))

我们还可以使用 sort_keys
参数按字母顺序对键进行排序,并将其设置为 True
。
print(json.dumps(fcc_data, indent=4, sort_keys=True))
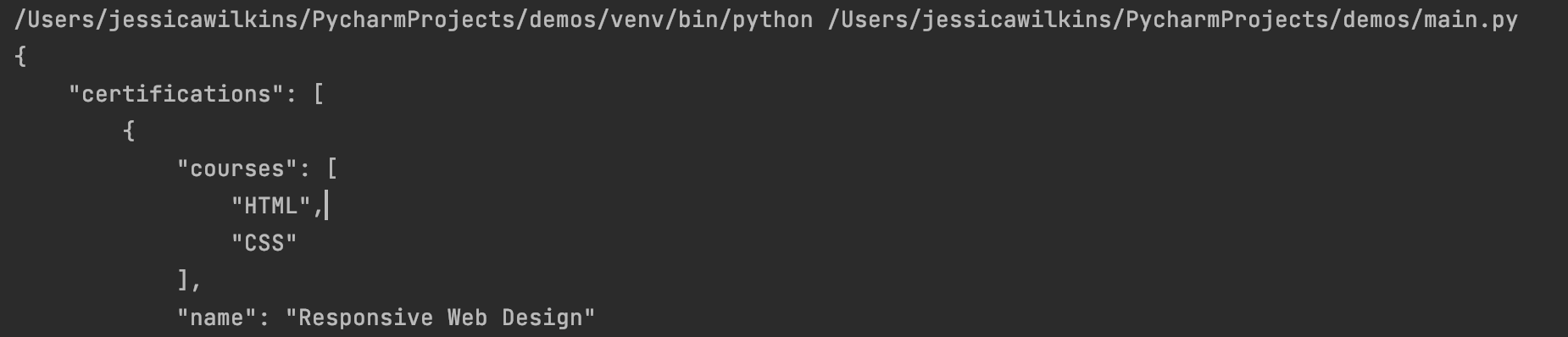
小结
JSON(JavaScript Object Notation 对象表示法)是一种流行的结构化数据的方式。它被用来在网络应用程序和服务器之间交换信息。
如果你需要解析一个返回字典的 JSON 字符串,那么你可以使用 json.load()
方法。
如果你需要解析一个返回字典的 JSON 文件,那么你可以使用 json.load()
方法。